SPA Layout
Program.cs
- Main
- Call CreateHostBuilder
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
- CreateHostBuilder
- Call Startup class
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
Startup.cs
- Startup
- Call
_Host.cshtml
- Call
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
...
app.UseEndpoints(endpoints =>
{
endpoints.MapBlazorHub();
endpoints.MapFallbackToPage("/_Host");
});
}
_Host.cshtml
- Call
App.razor
<app>
<component type="typeof(App)" render-mode="ServerPrerendered" />
</app>
App.razor
- define layout in
DefaultLayout
- Call
MainLayout.razor
<RouteView RouteData="@routeData" DefaultLayout="@typeof(MainLayout)" />
- Router Componenet
- AppAssembly: Gets or sets the assembly that should be searched for components matching the URI.
- Found: Gets or sets the content to display when a match is found for the requested route.
- NotFound: Gets or sets the content to display when no match is found for the requested route.
public class Router : IComponent, IHandleAfterRender, IDisposable
{
public Router();
...
[Parameter]
public Assembly AppAssembly { get; set; }
[Parameter]
public RenderFragment<RouteData> Found { get; set; }
[Parameter]
public RenderFragment NotFound { get; set; }
public void Attach(RenderHandle renderHandle);
public void Dispose();
public Task SetParametersAsync(ParameterView parameters);
}
MainLayout.razor
- Call
NavMenu.razor
<div class="sidebar">
<NavMenu />
</div>
- Call
Body
<div class="content px-4">
@Body
</div>
- Body Property
- Gets the content to be rendered inside the layout.
public RenderFragment Body { get; set; }
NavMenu.razor
- Redirect Web
- For mobile, side Menu can be a toggle
<div class="top-row pl-4 navbar navbar-dark">
<a class="navbar-brand" href="">BlazorSPA</a>
<button class="navbar-toggler" @onclick="ToggleNavMenu">
<span class="navbar-toggler-icon"></span>
</button>
</div>
Rout
URI
- Pages\ _Host.cshtml
- define how to rout
<base href="~/" />
Navigation
- NavigationManager
- You can use not only
<a>
Tag, but alsoNavigationManager
- Navigates to the specified URI
- You can use not only
public void NavigateTo(string uri, bool forceLoad = false);
- Pages\Counter.razor
- Use
Defendency Injection
- Use
@page "/counter"
...
@inject NavigationManager navManager
...
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
<a class="btn btn-primaty" href="FetchData">Go FetchData</a>
@code {
private void IncrementCount()
{
CurrentCount++;
navManager.NavigateTo("FetchData");
}
...
}
URI Utilizing
-
@page
- You can use URI components value
@page "/counter"
@page "/counter/{CurrentCount:int}"
...
<p>Current count: @CurrentCount</p>
...
@code {
private void IncrementCount()
{
CurrentCount++;
navManager.NavigateTo("FetchData");
}
[Parameter]
public int CurrentCount { get; set; }
}
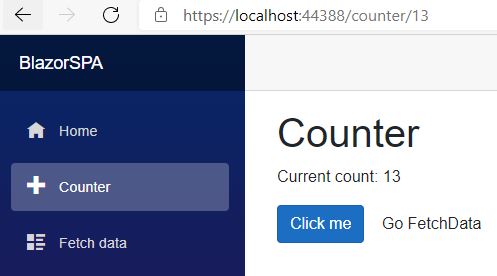
Page Layout
MainLayout.razor
- Base Layout in Blazor
@inherits LayoutComponentBase
- LayoutComponentBase
- Optional base class for components that represent a layout.
- Alternatively, components may implement Microsoft.AspNetCore.Components.IComponent directly and declare their own parameter named Microsoft.AspNetCore.Components.LayoutComponentBase.Body.
public abstract class LayoutComponentBase : ComponentBase
Layout Customizing
- Shared
- Create new layout by razor component
- link this layout in razor component of
Pages
by@layout
- Shared\MainLayout2.razor
...
<a href="https://docs.microsoft.com/aspnet/" target="_blank">Layout2</a>
...
- Pages\Counter.razor
...
@layout MainLayout2
...
- Pages
- link this layout in
[folder]\_imports.razor
by@layout
- It changes all layout of the folder
- Pages_imports.razor
- link this layout in
@layout MainLayout2
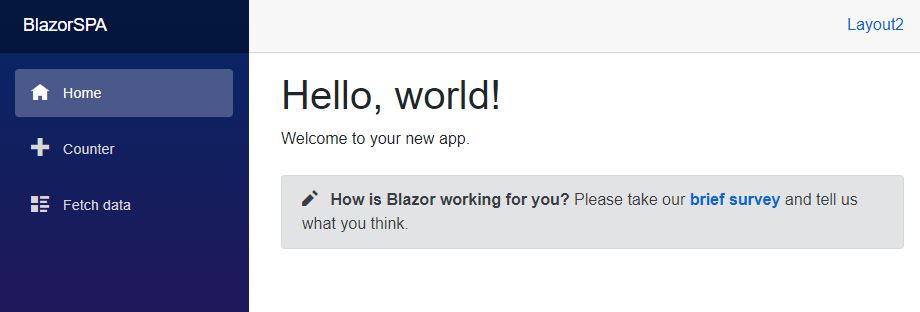
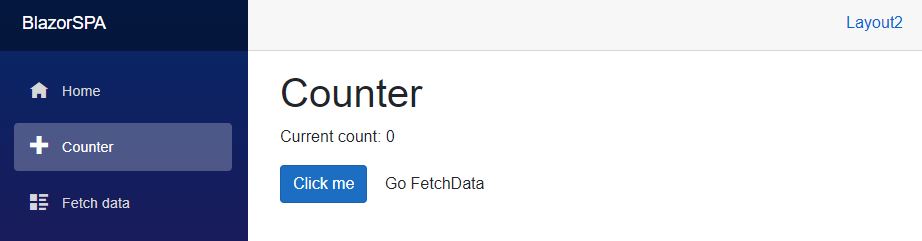
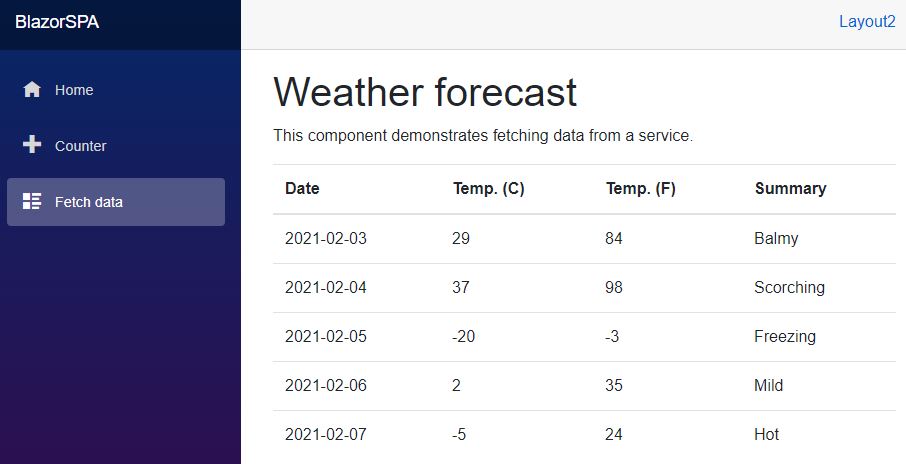