Pages
- M
- Model
- defines Data
- VC(or VM)
- View and Controller(or Model)
- defines UI
- defines Action
Code
- Models\HelloMessage.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace HelloEmptyRazor.Models
{
public class HelloMessage
{
public string Message { get; set; }
}
}
- Views\Index.cshtml
@page
@model HelloEmptyRazor.Pages.IndexModel
<html>
<head>
<title>Hello Razor Pages</title>
</head>
<body>
<h1>@Model.HelloMsg.Message</h1>
<hr />
<h2>@Model.Noti</h2>
<form method="post">
<label asp-for="HelloMsg.Message">Enter Message</label>
<br />
<input type="text" asp-for="HelloMsg.Message" />
<br />
<button type="submit">Submit</button>
</form>
</body>
</html>
- Views\Index.cshtml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using HelloEmptyRazor.Models;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
namespace HelloEmptyRazor.Pages
{
public class IndexModel : PageModel
{
[BindProperty]
public HelloMessage HelloMsg { get; set; }
public string Noti { get; set; }
public void OnGet()
{
this.HelloMsg = new HelloMessage()
{
Message = "Hello Razor Pages"
};
}
public void OnPost()
{
this.Noti = "Message Changed";
}
}
}
- Views_ViewImports.cshtml
@using HelloEmptyRazor
@using HelloEmptyRazor.Models
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
- Startup.cs
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace HelloEmptyRazor
{
public class Startup
{
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
}
}
}
}
Differnce with MVC
- Index.cshtml
- Razor Pages doesn’t have
asp-controller
andasp-action
, because it doesn’t have a controller
- Razor Pages doesn’t have
<form method="post">
- Startup.cs
- Razor Pages serves by Pages.
- Routing Method: Relative Path
To show a page, it search [Pages] folder and following path with filename
services.AddRazorPages();
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
}
Result
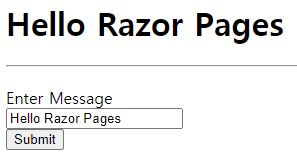
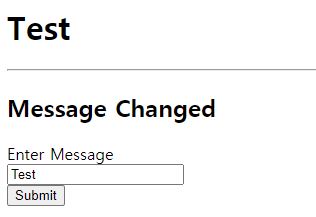