Code
using System;
namespace RockScissorsPaper
{
enum Choice
{
Rock = 0,
Scissors = 1,
Paper = 2
}
class Program
{
static void Main(string[] args)
{
Random rand = new Random();
int aiChoice = rand.Next(0, 3);
int choice = Convert.ToInt32(Console.ReadLine());
switch (choice)
{
case (int)Choice.Rock:
Console.WriteLine("Your choice is rock.");
break;
case (int)Choice.Scissors:
Console.WriteLine("Your choice is scissors.");
break;
case (int)Choice.Paper:
Console.WriteLine("Your choice is paper.");
break;
}
switch (aiChoice)
{
case (int)Choice.Rock:
Console.WriteLine("AI choice is rock.");
break;
case (int)Choice.Scissors:
Console.WriteLine("AI choice is scissors.");
break;
case (int)Choice.Paper:
Console.WriteLine("AI choice is paper.");
break;
}
if(choice == (int)Choice.Rock)
{
if(aiChoice == (int)Choice.Rock)
{
Console.WriteLine("Draw :|");
}
else if(aiChoice == (int)Choice.Scissors)
{
Console.WriteLine("Win :)");
}
else
{
Console.WriteLine("Lose :(");
}
}
else if(choice == (int)Choice.Scissors)
{
if (aiChoice == (int)Choice.Rock)
{
Console.WriteLine("Lose :(");
}
else if (aiChoice == (int)Choice.Scissors)
{
Console.WriteLine("Draw :|");
}
else
{
Console.WriteLine("Win :)");
}
}
else
{
if (aiChoice == (int)Choice.Rock)
{
Console.WriteLine("Win :)");
}
else if (aiChoice == (int)Choice.Scissors)
{
Console.WriteLine("Lose :(");
}
else
{
Console.WriteLine("Draw :|");
}
}
}
}
}
Static
- static
- Can be used without invoking an instance
- Stack Memory
static void Main(string[] args)
new className()
- new Operator
- Create an object and call a constructor
- Heap Memory
Random rand = new Random();
Random
- Exist in System Namespace
- Random Constructor
- Use
new
keyword and the constructor to create Random type object
- Use
Random rand = new Random();
Next() Method
- Next(int minValue, int maxValue)
- minValue ~ maxValue-1
- Return Integer(n>=0)
- Next(int maxValue)
- 0 ~ maxValue-1
Type Casting
- String -> Int
- Convert.ToInt32(String string)
- If string is null, then 0
int choice = Convert.ToInt32(Console.ReadLine());
- enum -> int
- (Type)enumClass.enumObject
- Be Careful when you change type bigger to small
switch (choice)
{
case (int)Choice.Rock:
Console.WriteLine("Your choice is rock.");
break;
case (int)Choice.Scissors:
Console.WriteLine("Your choice is scissors.");
break;
case (int)Choice.Paper:
Console.WriteLine("Your choice is paper.");
break;
}
Console.ReadLine()
- Input string from console
int choice = Convert.ToInt32(Console.ReadLine());
Console.WriteLine()
- Print string on console
Console.WriteLine("Your choice is rock.");
Result
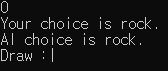
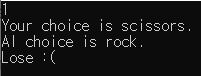
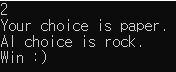