Code
- Program.cs
using System;
namespace TextRPG2
{
class Program
{
static void Main(string[] args)
{
Game game = new Game();
while(true){
game.Process();
}
}
}
}
- Player.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace TextRPG2
{
public enum PlayerType
{
None = 0 ,
Knight = 1,
Archer = 2,
Mage = 3
}
class Player : Creature
{
protected PlayerType type = PlayerType.None;
protected Player(PlayerType type) : base(CreatureType.Player)
{
this.type = type;
}
public PlayerType GetPlayerType() { return type; }
}
class Knight : Player
{
public Knight() : base(PlayerType.Knight)
{
SetInfo(100, 10);
}
}
class Archer : Player
{
public Archer() : base(PlayerType.Archer)
{
SetInfo(75, 12);
}
}
class Mage : Player
{
public Mage() : base(PlayerType.Mage)
{
SetInfo(50, 15);
}
}
}
- Monster.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace TextRPG2
{
public enum MonsterType
{
None = 0,
Slime = 1,
Orc = 2,
Skeleton = 3
}
class Monster : Creature
{
protected MonsterType type = MonsterType.None;
protected Monster(MonsterType type) : base(CreatureType.Monster)
{
this.type = type;
}
public MonsterType GetMonsterType() { return type; }
}
class Slime : Monster
{
public Slime() : base(MonsterType.Slime)
{
SetInfo(10, 10);
}
}
class Orc : Monster
{
public Orc() : base(MonsterType.Orc)
{
SetInfo(20, 15);
}
}
class Skeleton : Monster
{
public Skeleton() : base(MonsterType.Skeleton)
{
SetInfo(15, 20);
}
}
}
- Creature.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace TextRPG2
{
public enum CreatureType
{
None,
Player = 1,
Monster = 2,
}
class Creature
{
CreatureType type;
protected Creature(CreatureType type)
{
this.type = type;
}
protected int hp = 0;
protected int attack = 0;
public void SetInfo(int hp, int attack)
{
this.hp = hp;
this.attack = attack;
}
public int GetHp() { return hp; }
public int GetAttack() { return attack; }
public bool IsDead() { return hp <= 0; }
public void OnDamaged(int damage)
{
hp -= damage;
if (hp < 0)
hp = 0;
}
}
}
- Game.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace TextRPG2
{
public enum GameMode
{
None,
Lobby,
Town,
Field
}
class Game
{
private GameMode mode = GameMode.Lobby;
private Player player = null;
private Monster monster = null;
private Random rand = new Random();
public void Process()
{
switch (mode)
{
case GameMode.Lobby:
ProcessLobby();
break;
case GameMode.Town:
ProcessTown();
break;
case GameMode.Field:
ProcessField();
break;
}
}
public void ProcessLobby()
{
Console.WriteLine("Select Type");
Console.WriteLine("[1] Knight");
Console.WriteLine("[2] Archer");
Console.WriteLine("[3] Mage");
string input = Console.ReadLine();
switch (input)
{
case "1":
player = new Knight();
mode = GameMode.Town;
break;
case "2":
player = new Archer();
mode = GameMode.Town;
break;
case "3":
player = new Mage();
mode = GameMode.Town;
break;
}
}
public void ProcessTown()
{
Console.WriteLine("You entered Town!");
Console.WriteLine("[1] Go to Field");
Console.WriteLine("[2] Go to Lobby");
string input = Console.ReadLine();
switch (input)
{
case "1":
mode = GameMode.Field;
break;
case "2":
mode = GameMode.Lobby;
break;
}
}
public void ProcessField()
{
Console.WriteLine("You entered Field!");
Console.WriteLine("[1] Fight");
Console.WriteLine("[2] Run away to town with a certain chance");
CreateRandomMonster();
string input = Console.ReadLine();
switch (input)
{
case "1":
ProcessFight();
break;
case "2":
TryExcape();
break;
}
}
private void TryExcape()
{
int randValue = rand.Next(0, 101);
if(randValue < 33)
{
mode = GameMode.Town;
}
else
{
ProcessFight();
}
}
private void ProcessFight()
{
while (true)
{
int damage = player.GetAttack();
monster.OnDamaged(damage);
if (monster.IsDead())
{
Console.WriteLine("You Win! :)");
Console.WriteLine($"Left HP : {player.GetHp()}");
break;
}
damage = monster.GetAttack();
player.OnDamaged(damage);
if (player.IsDead())
{
Console.WriteLine("You Lose! :(");
mode = GameMode.Lobby;
break;
}
}
}
private void CreateRandomMonster()
{
int randValue = rand.Next(0, 3);
switch (randValue)
{
case 0:
monster = new Slime();
Console.WriteLine("Slime is created!");
break;
case 1:
monster = new Orc();
Console.WriteLine("Orc is created!");
break;
case 2:
monster = new Skeleton();
Console.WriteLine("Skeleton is created!");
break;
}
}
}
}
Class className : parentClassName
- Inheritance
- inheritance of the characteristics of the parent concept
class Player : Creature
Access Modifier
- public
- Can access the member from anywhere
public enum PlayerType
- protected
- Not accessible from outside the class
- Accessible from the derived class
protected PlayerType type = PlayerType.None;
- private
- Accessible only from within the class.
private GameMode mode = GameMode.Lobby;
base & this
- base()
- Access members of the base class within the derived class
protected Player(PlayerType type) : base(CreatureType.Player)
- this
- Point to the current instance of the class
this.type = type;
Constructor
- Constructor
- A method whose name is the same as the name of that type
- The method signature contains only a list of method names and parameters and does not include a return type
- To set defaults, limit instantiation, and write flexible, readable code
class Player : Creature
{
protected Player(PlayerType type) : base(CreatureType.Player)
{
this.type = type;
}
...
Result
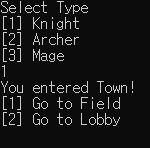
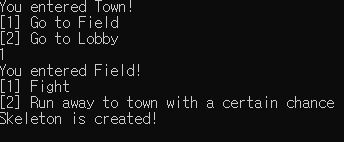
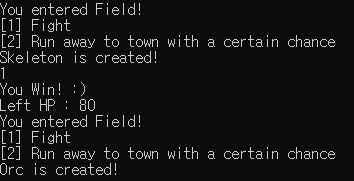