IDE
Download
- Download MongoDB for VSCode in Visual Studio Code

Create Cluster
- Create Cluster on MongoDB Web
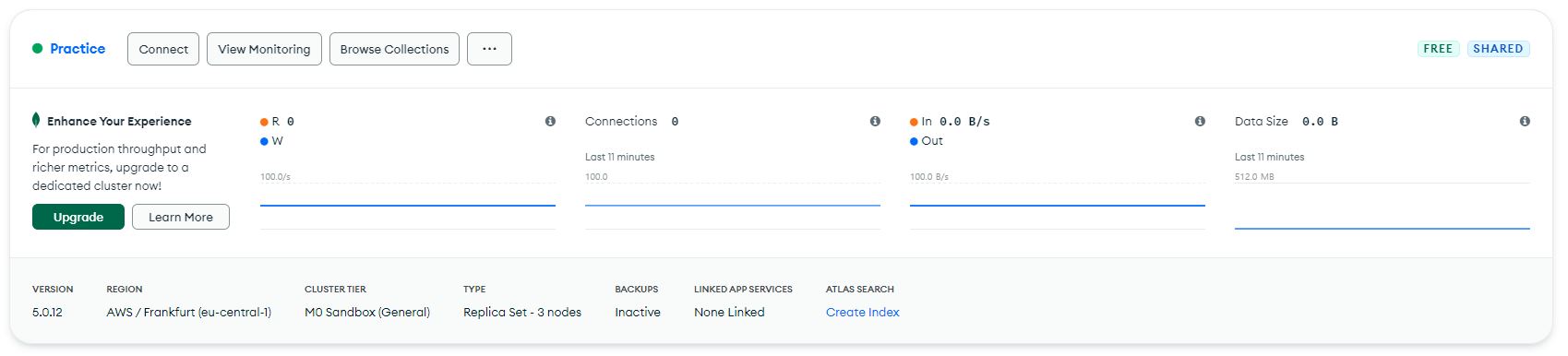
Connection
- Click Connection button on Mongo DB web and check your cluster connection string.
- Click MongoDb Playground on side bar.
- Connect your cluster in VSCode with your connection string.
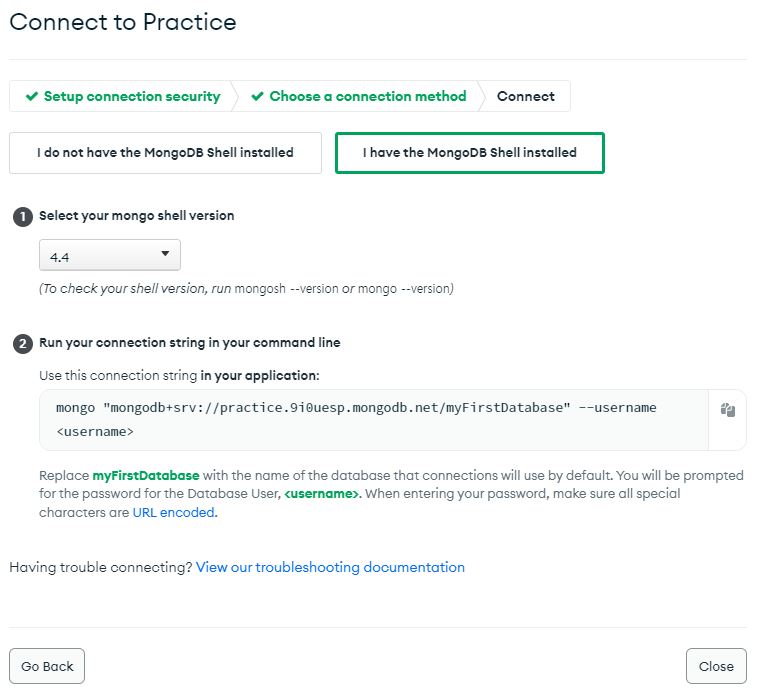
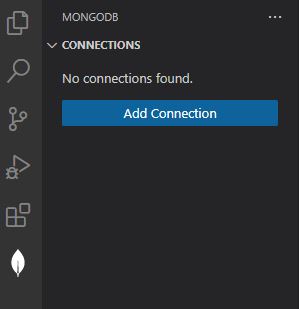
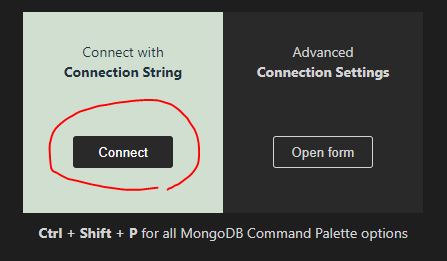
- Change the name
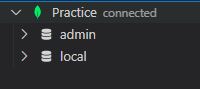
Playground
- Create new Playground to add your script.
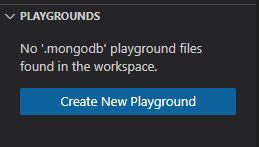
IRUD
Insert
use
switchs current database.db.{collection}
accesses to the collection.insertOne
inserts one document on your collection.
use('video');
var movie = {"title" : "Star Wars: Episode IV - A New Hope" ,
"director" : "George Lucas",
"year" : 1977};
db.movies.insertOne(movie);
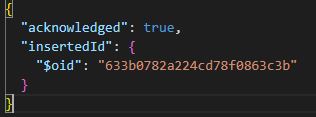
insertMany
inserts many document on your collection.
db.movies.insertMany([{"title" : "Ghostbusters"},
{"title" : "E.T"},
{"title" : "Blade Runner"}]);
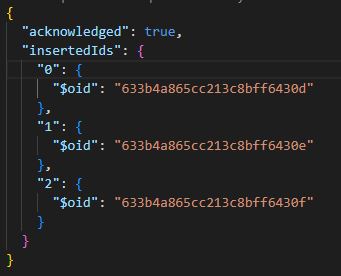
Read
find
shows all documents.
db.movies.find().pretty();
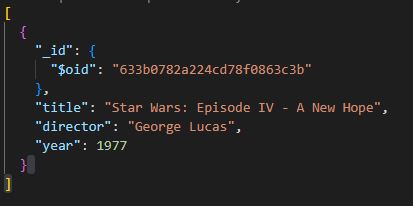
findOne
shows only one document.
db.movies.findOne();
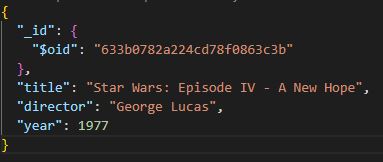
Update
updateOne
updates one document.
db.movies.updateOne({title : "Star Wars: Episode IV - A New Hope"},
{$set : {reviews:[]}});
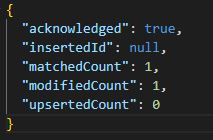
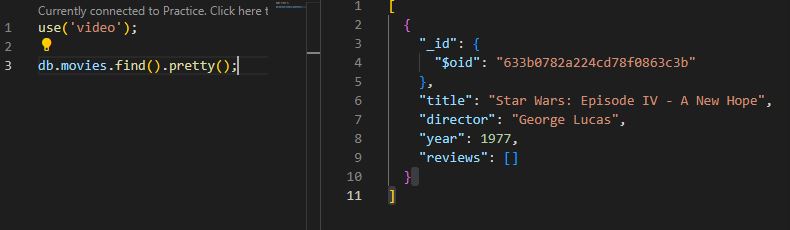
Delete
deleteOne
deletes one document.
db.movies.deleteOne({title : "Star Wars: Episode IV - A New Hope"});
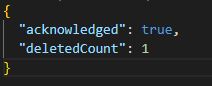
deleteMany
deletes many documents.
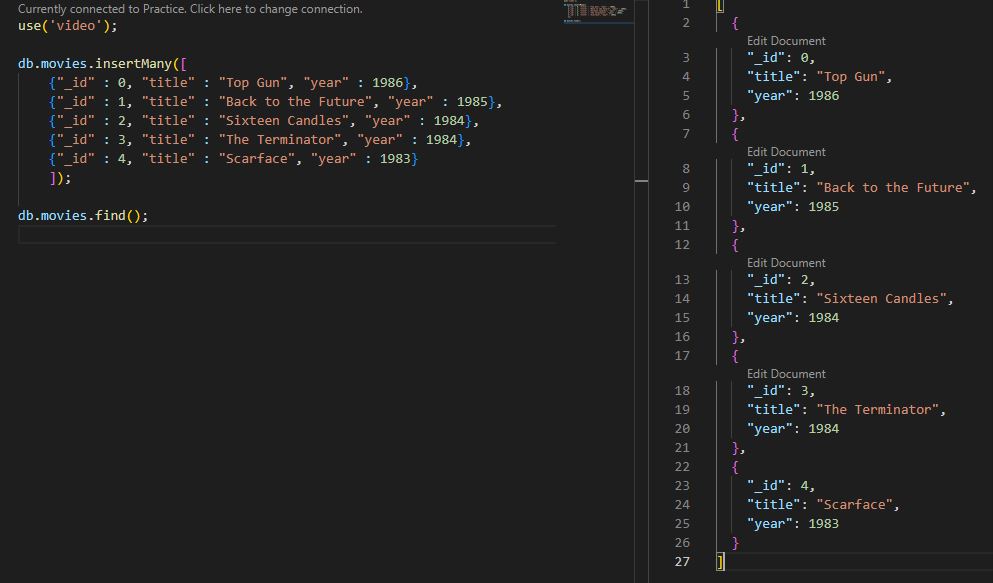
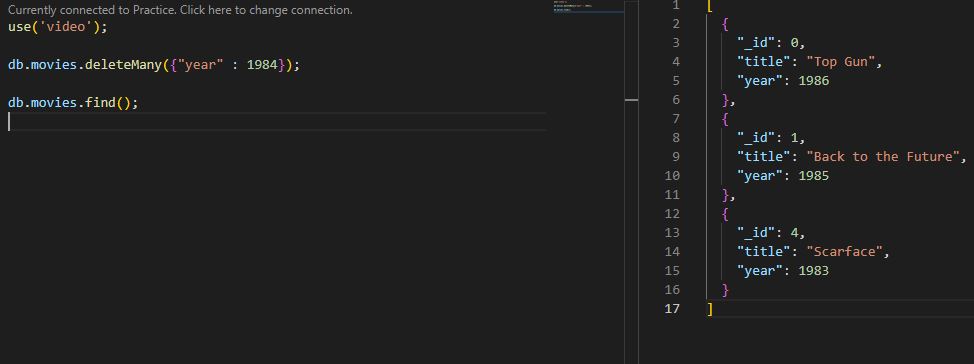
deleteMany({})
deletes all documents.

Drop
drop
drops the collection.
Blocking
db.dropDatabase = DB.prototype.dropDatabase = no; // block to delete database
DBCollection.prototype.drop = no; // block to delete collection
DBCollection.prototype.dropIndex = no; // block to delete Index
DBCollection.prototype.dropIndexes = no; // block to delete Indexes
Document
Replace
replaceOne
changes database version.
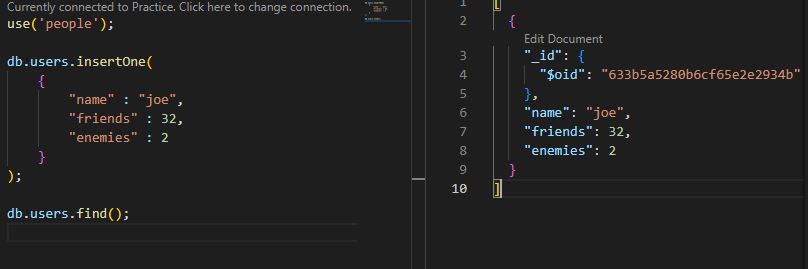
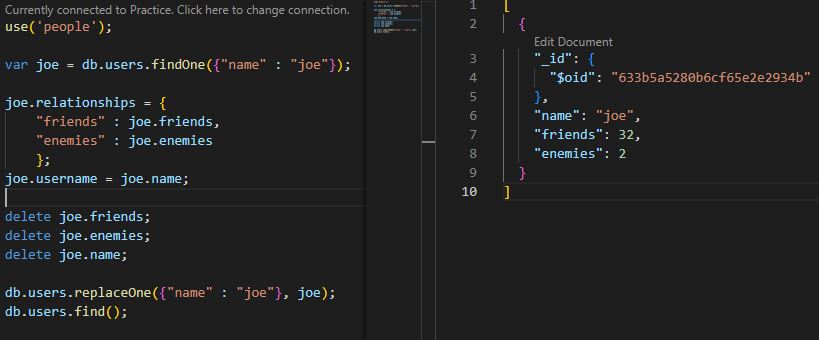
Modifier
$inc
increase field value.
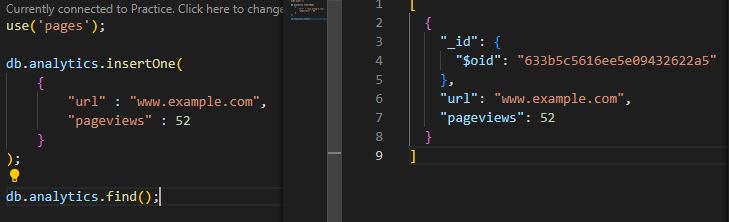
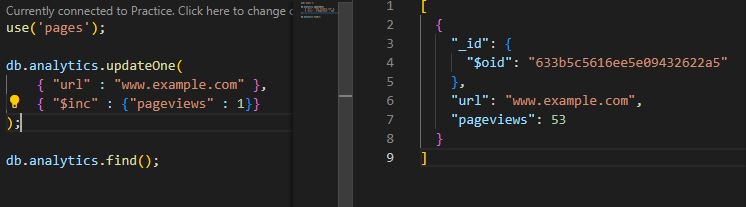
$set
set field value.$unset
remove field from the document.
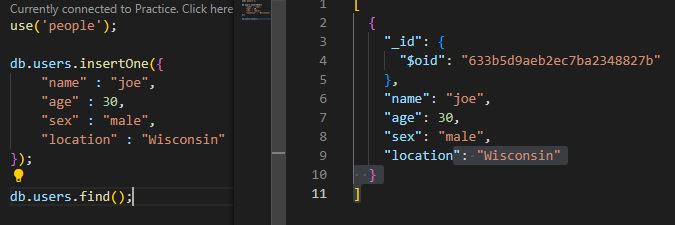
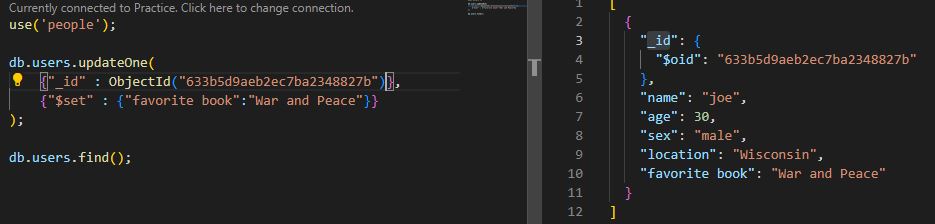
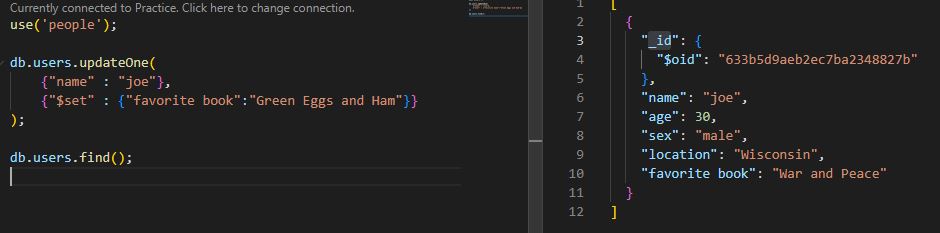
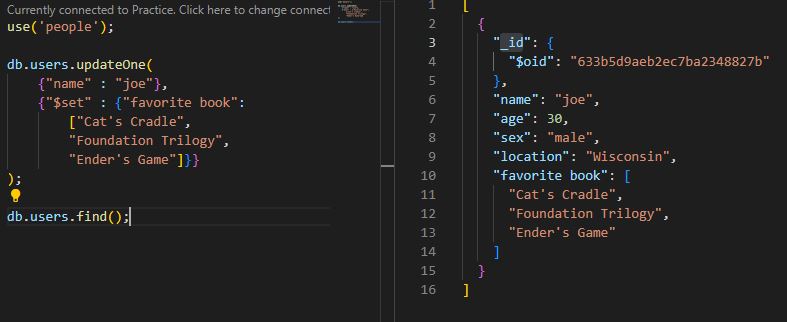
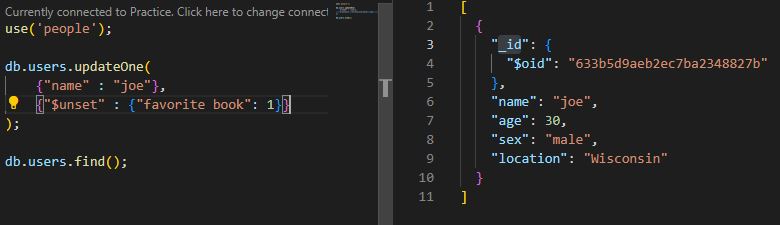
$push
adds element or create new array.$each
adds elements at once.$slice
limits the array size.$sort
sorts elements with the field value.$each
is always needed when you use$push
with$slice
or$sort
.
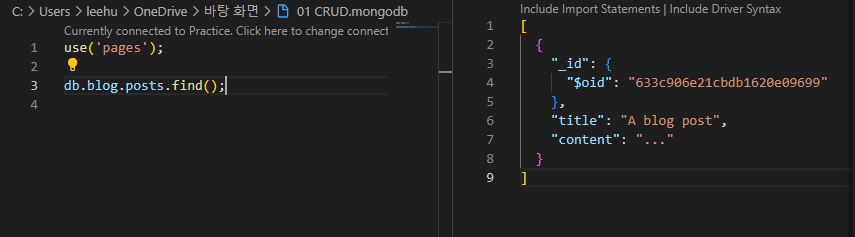
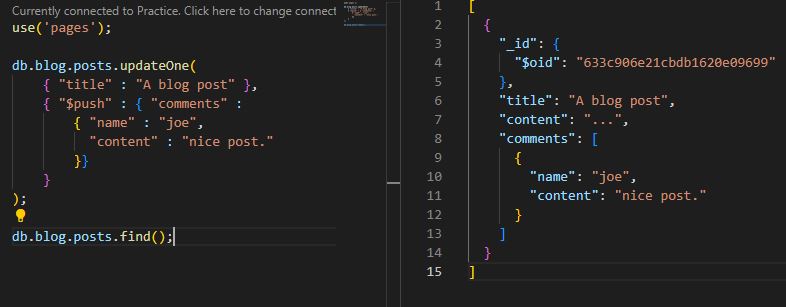
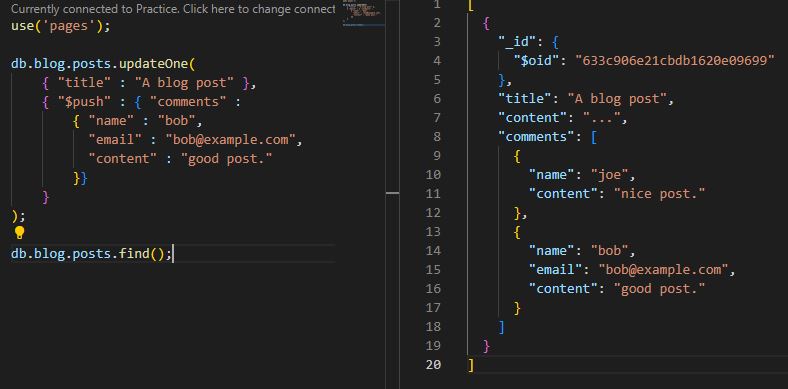
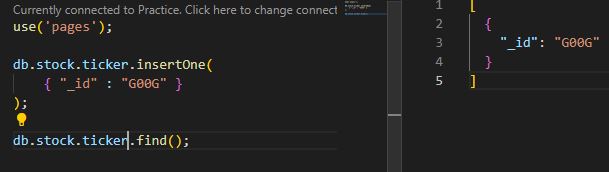
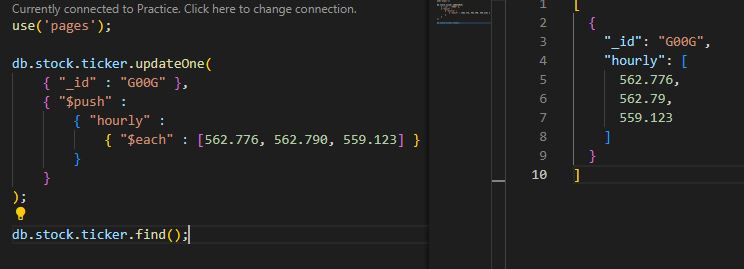
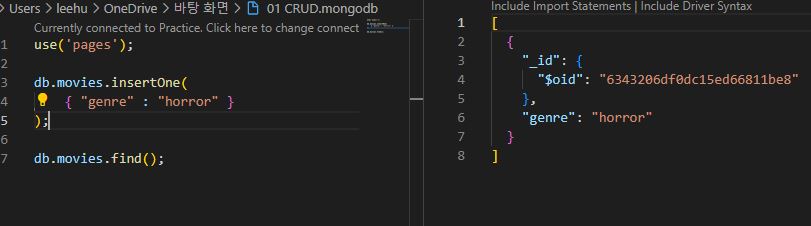
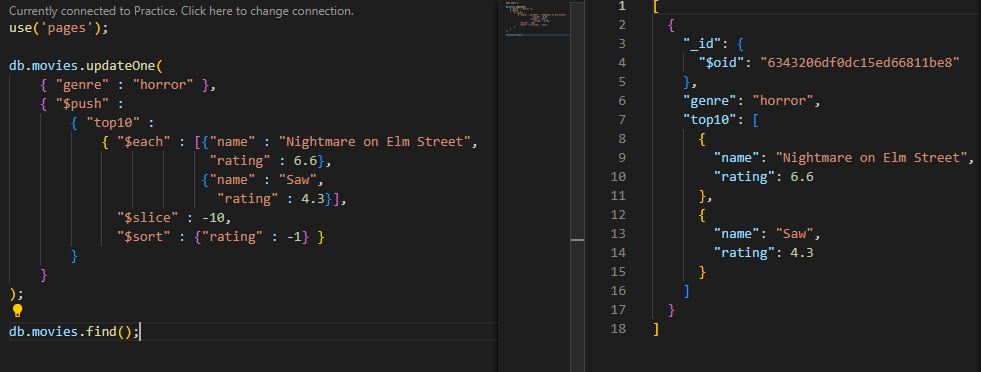
$ne
finds documents which don’t have not specific value.
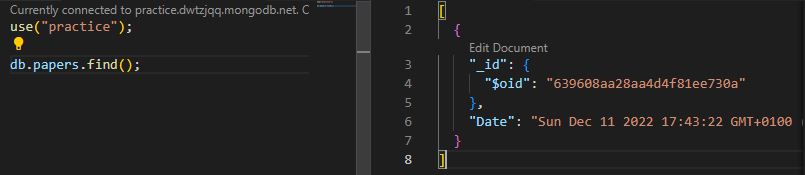
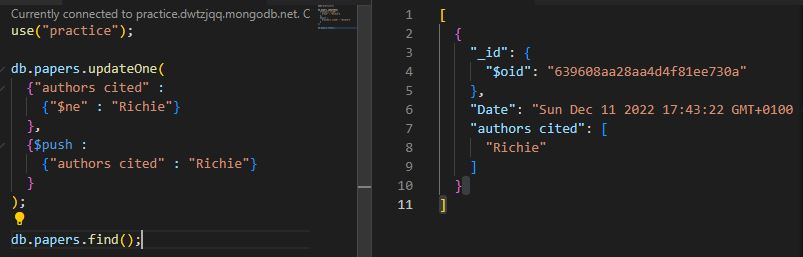
$addToSet
add specific value without duplication.
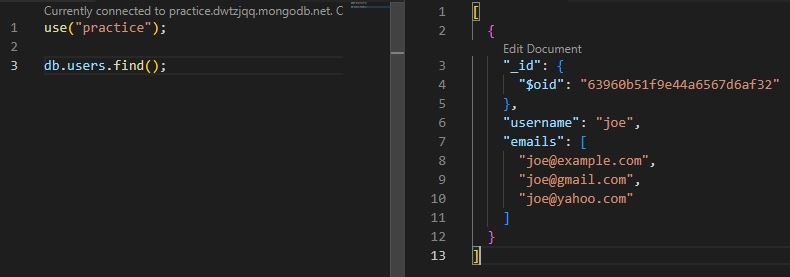
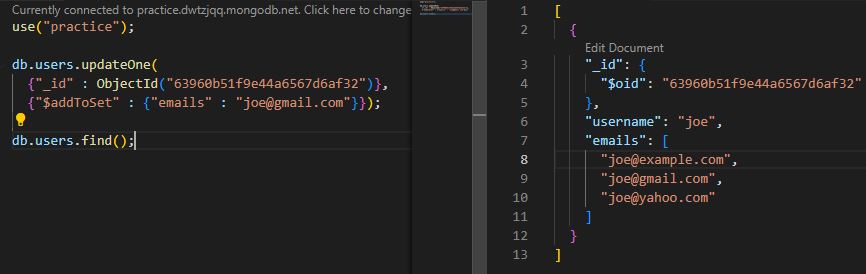
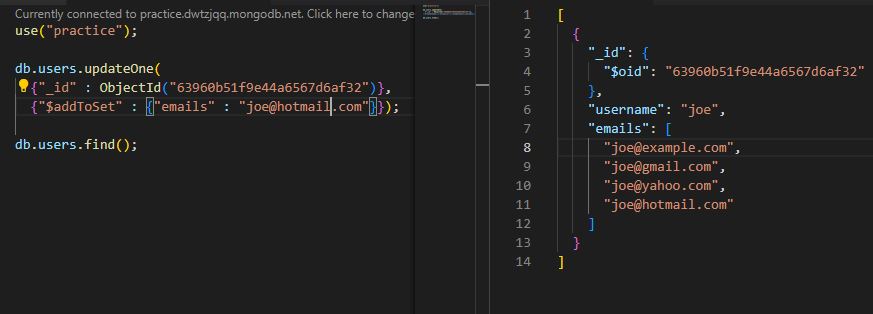
$ne/$push
can add only one value and$addToSet/$each
can add several values at once.
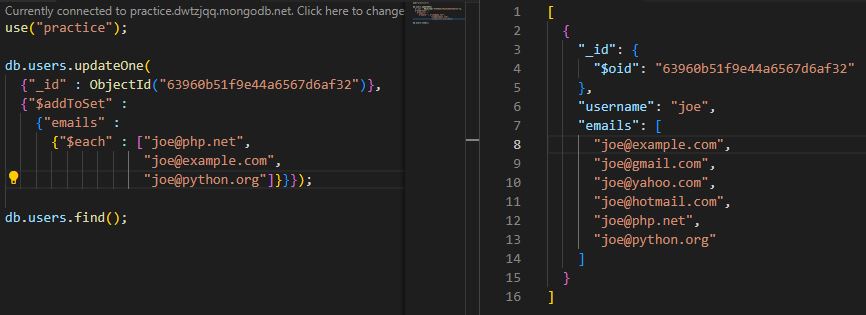
$pop
removes array elements like stack or queue.{"$pop" : {"key" : 1}}
removes elements from last.{"$pop" : {"key" : -1}}
removes elements from first.
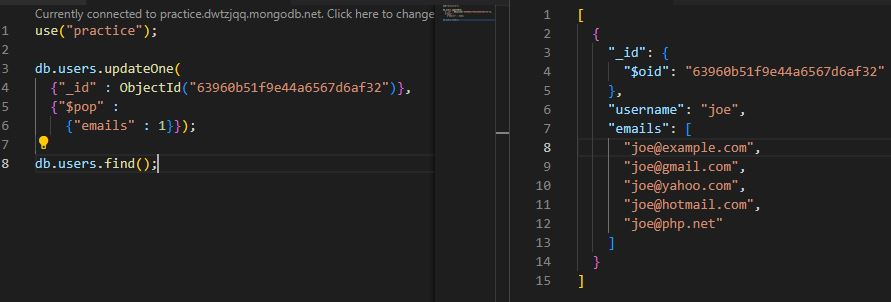
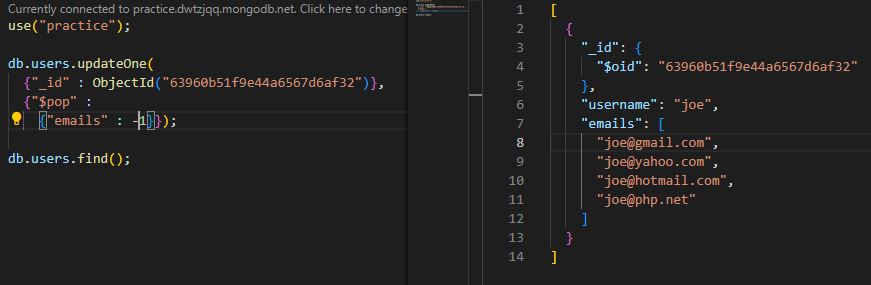
$pull
removes array elements with specific conditions.
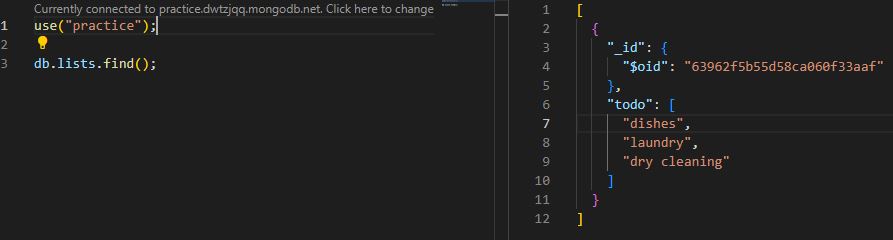
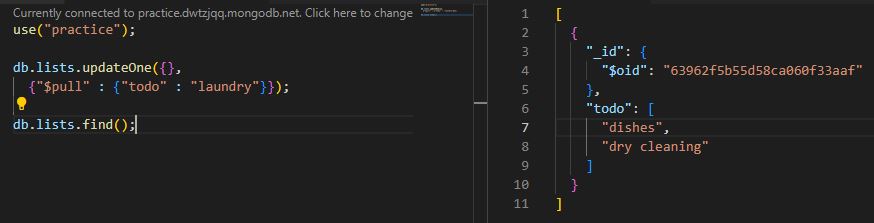