Game design
- Maze
- Background
- Ball
- Trap
- Goal
- Title
- Clear
Create maze and background
- Create floor
- Create Cube and change Name to
Floor
- Change Scale to (20, 1, 20)
Maze Floor - Create Physic Material and drag and drop to
Floor
- Change Dynamic Friction and Static Friction to 0.2
Maze Floor - Create Cube and change Name to
- Create Point Light
- Delete Directional Light, create Point Light
- Change Rosition to (0,12,0), Lange to 50
Maze Floor - Paint floor
- Save an image to
Assets
folder
Maze Floor - Drag and drop image to
Floor
object of hierarchy
- Save an image to
- Change position of Camera
- Change Position to (0,1,-10)
Maze Floor - Create background
- Chagne Position to (0,1,-10)
- Put black color on background
- Create Plane, Change name to
BG
, and change Scale to (6,1,6) and Position to (0,0,0)
Maze Floor
Create walls and barriers
- Create outer walls
- Create Cube and change Name to
Wall
- Take same Material with
Floor
, and change Position to (0,1,-10) and Scale to (20,1,1)

- Create Prefab and chang Name to
wall
- Drag and Drop
wall
in hierarchy to Prefab
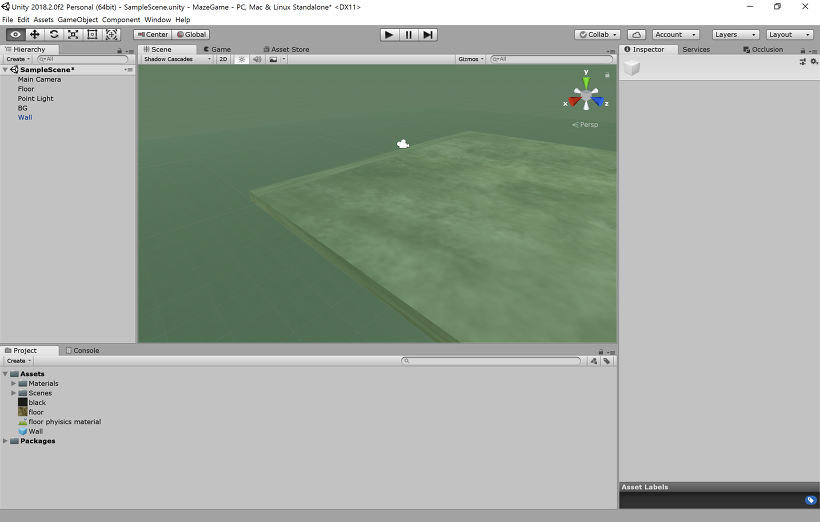
- Drag and Drop
wall
of Project to Hierarchy - Change Position
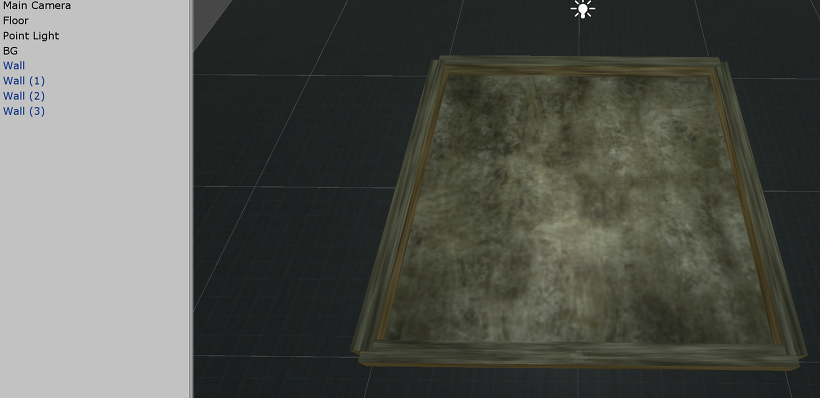
- Create barriers
- Create Prefab and change Name to
barrier
- Create Cube and change Position to (0,1,0)

- Make barriers
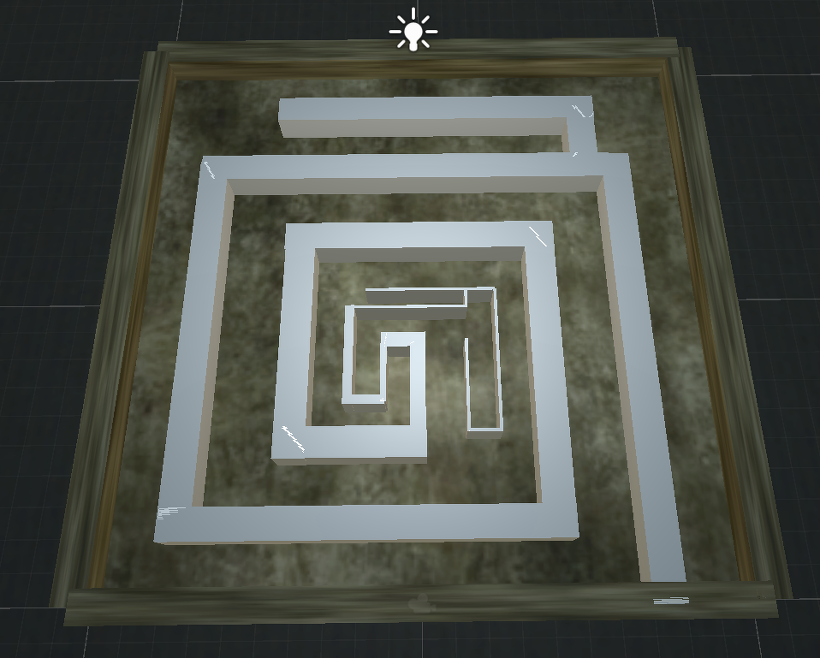
- Create Material
- Save a white image to
Assets
folder
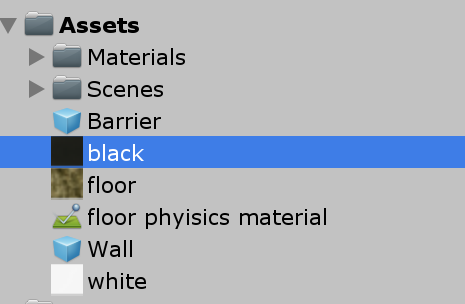
- Put the texture to
barrier
- Change Color in Inspector
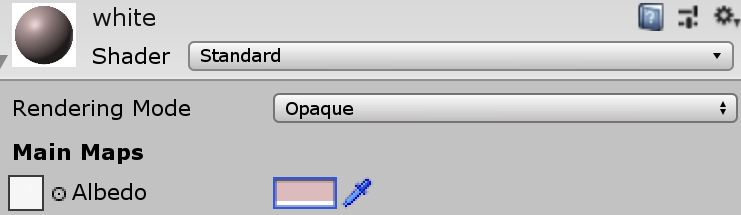
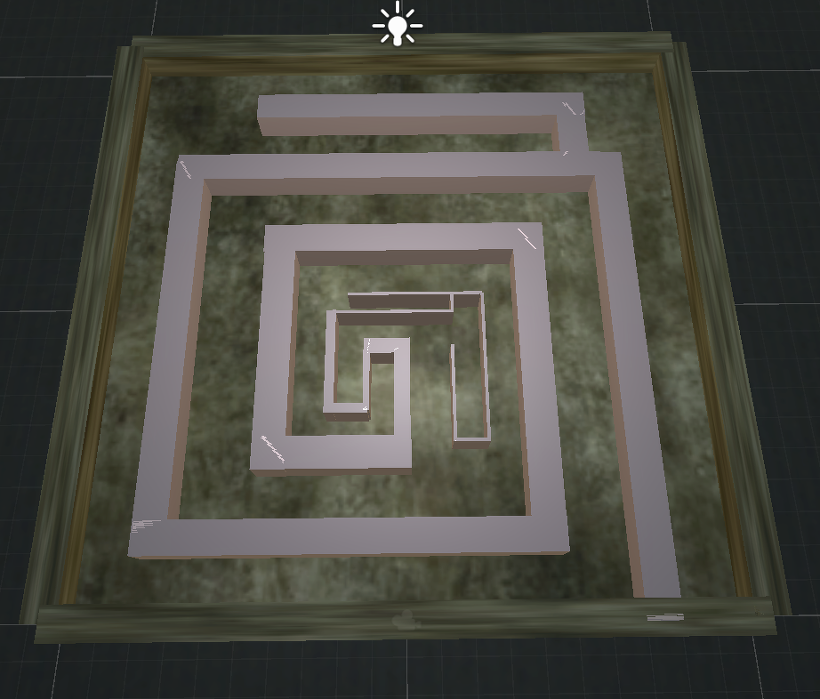
- Change background Position to (0,-100,0), and Scale to (100,1,100)
- Change Position of Main Camera
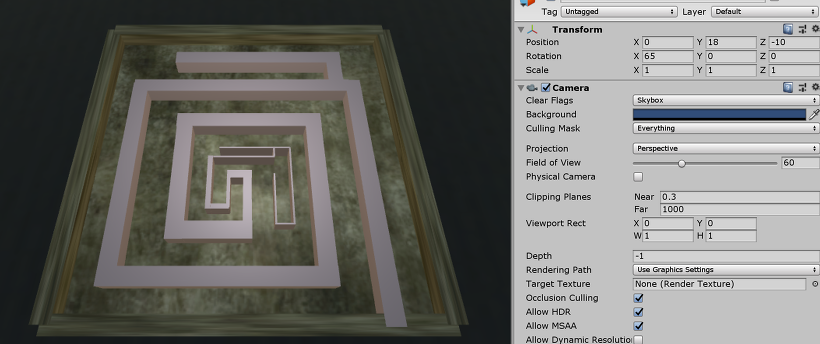
- Create Empty Game Object, and change Position to (0,0,0)
- Put
wall
s,floor
, andbarrier
s to under the Object
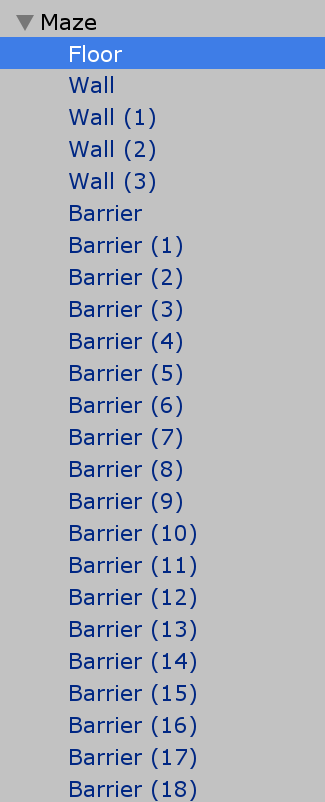
Create a ball
Create control script
GravityController.js
function Update() {
Physics.gravity = Quaternion.AngleAxis(Input.GetAxis("Horizontal") * 60.0, Vector3.forward) * Quaternion.AngleAxis(Input.GetAxis("Vertical") * -60.0, Vertor3.right) * (Vector3.up * -20.0);
}
- Create empty game object and change Name to
Gravity Controller
-
Drag and Drop GravityController script to Gravity Controller object
Ball.js
function Update() {
rigidbody.WakeUp();
}
Rig
- Tilt Rig
- Create Empty Game Object and change Name to
Tilt Rig
- Change Position to (0,0,0)
- Put Main Camera,
BG
, andPoint Light
to under theTilt Rig
- Create Empty Game Object and change Name to
TiltRig.js
function Update() {
transform.rotation = Quaternion.AngleAxis(Input.GetAxis("Horizontal") * 10.0, Vector3.forward) * Quaternion.AngleAxis(Input.GetAxis("Vertical") * -10.0, Vector3.right);
}
-
Drag and Drop
TiltRig
Script toTilt Rig
Object -
Result
Create a trap
- Create warp zone
- Create Cube and change Name to
Trap
- Select
is Trigger
of Collider Componenet
Maze Trap - Create Cube and change Name to
- Create Tag
- Change Name to
ball
- Prefab
ball
and selectball
Tag in Inspector
Maze Trap - Change Name to
- Restart point
- Create Empty Game object and change Name to
Respawn Point
- Change Tag to
Respawn
Maze Trap - Create Empty Game object and change Name to
Trap.js
functionOnTriggerEnter(other:Collider) {
if (other.gameObject.tag == "Ball"){
var respawn : GameObject = GameObject.FindWithTag("Respawn");
other.gameObject.transform.position = respawn.transform.position;
}
}
- Drag and Drop Script to
Trap
Object
Create a goal
- Create goal Trigger
- Create Cube and change Name to
Goal
- Select
is Trigger
of Collider in Inspector - Create Material to paint and change Name to
Goal
Maze Goal - Create Cube and change Name to
Goal.js
private var ballCount : int;
private var counter : int;
private var cleared : boolean;
function Start () {
ballCount = GameObject.FindGameObjectsWithTag("Ball").length;
}
function OnTriggerEnter (other : Collider) {
if(other.gameObject.tag == "Ball"){
counter++;
if(cleared == false && counter == ballCount){
cleared = true;
Debug.Log("Cleared!");
}
}
}
function OnTriggerExit(other : Collider) {
if(other.gameObject.tag == "Ball") {
counter--;
}
}
Show clear message
Goal.js
(add)
function OnGUI() {
if (cleared == true){
var sw : int = Screen.width;
var sh : int = Screen.height;
GUI.Label(Rect(sw / 6, sh / 3, sw * 2 / 3, sh / 3), "CLEARED!");
}
}
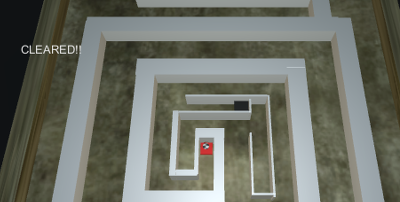
var labelStyle : GUIStyle;
Edit OnGUI()
function OnGUI() {
if (cleared == true){
var sw : int = Screen.width;
var sh : int = Screen.height;
GUI.Label(Rect(sw / 6, sh / 3, sw * 2 / 3, sh / 3), "CLEARED!", labelStyle);
}
}
- Change color, font, size and alignment in Inspector
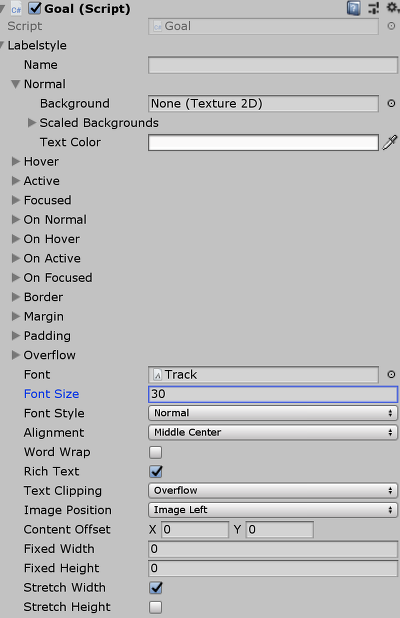
Create title scene
- Structure
Maze Title - Save
Main
Scene- File>Save Scene
- Change Name to
Main
- File>Build Settings…
- Press
Add Current
Maze Title - Create
Title
Scene- File> Save Scene As…
- Change name to
Title
- Add
Title
by PressAdd Current
to add inScene In Build
- Put
Title
in the Top
- Build
Title
Scene- Delete
Barrier
,Trap
andGoal
in Hierarchy View
Maze Title TitleScreen.js
- Delete
var labelStyle : GUIStyle;
function Update(){
if (Input.GetButtonDown("Jump")){
Application.LoadLevel("Main");
}
}
function OnGUI(){
var sw = Screen.width;
var sh = Screen.height;
GUI.Label(Rect(0, sh/4, sw, sh/4), "BALL MAZE", labelStyle);
GUI.Label(Rect(0, sh/2, sw, sh/4), "Hit Space Key", labelStyle);
}
- Create Empty Game Object and change Name to
Title Screen
- Drag and drop
TitleScreen.js
toTitle Screen
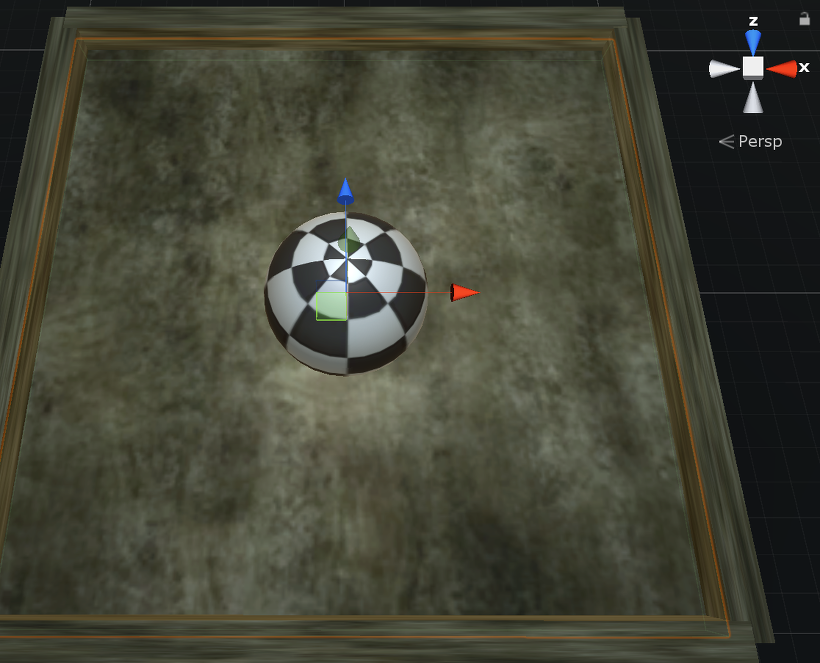
- Change action after game clear
Goal.js
(change)
function OnTriggerEnter (other : Collider) {
if(other.gameObject.tag == "Ball"){
counter++;
if(cleared == false && counter == ballCount){
cleared = true;
// here
yield WaitForSecond(2.0);
Application.LoadLevel("Title");
}
}
}
Improve presentation
- Change lighting
Edit
>Render Settings
- Click
Ambient Light
- Set
Color
andBrightness
- Set ball Specular
- Choose
Specular
inShader
in Inspector ofBall
- Set
Shininess
- Choose
- Set flicker effect on
Trap
FlickerEffect.js
private var originalColor : Color;
function Start(){
originalColor = renderer.material.color;
}
function Update(){
var level : float = Mathf.Abs(Mathf.Sin(Time.time * 20));
renderer.material.color = originalColor * level;
}
- Drag and drop
FlickerEffect.js
toTrap
prefab