Vue Install
- open terminal and type
npm init vue@latest
to create vue project
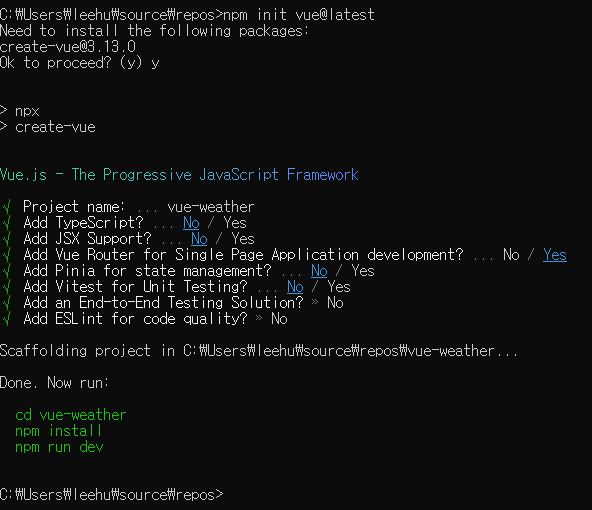
Extensions
- Vue Language Features (Volar)
- Vue VSCode Snippets
- Tailwind CSS IntelliSense
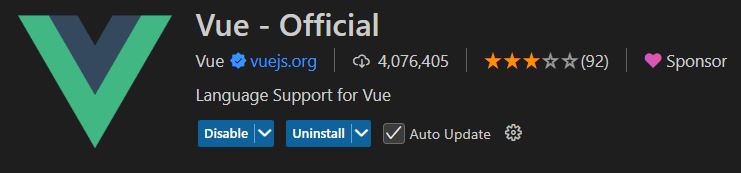
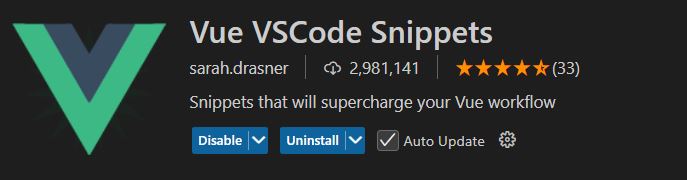
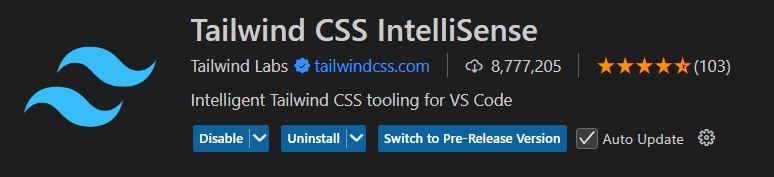
Prepare
- remove all files and folders in components folder
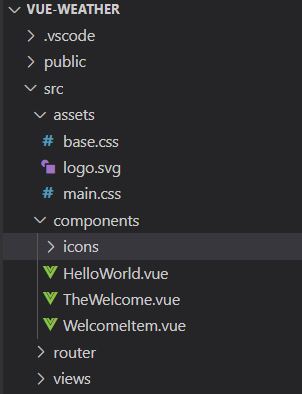
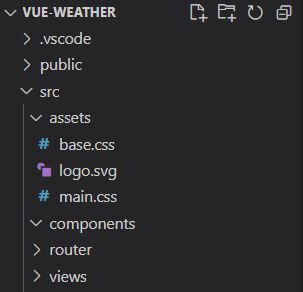
- remove all files in assets folder
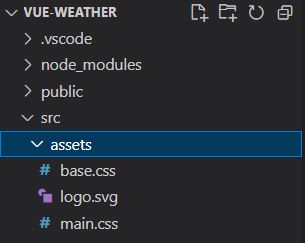
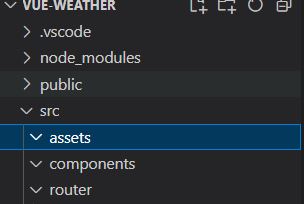
- open index.js in router folder
-
remove about url
- index.js
import { createRouter, createWebHistory } from 'vue-router'
import HomeView from '../views/HomeView.vue'
const router = createRouter({
history: createWebHistory(import.meta.env.BASE_URL),
routes: [
{
path: '/',
name: 'home',
component: HomeView,
}
],
})
export default router
- delete
AboutView.vue
file in views folder
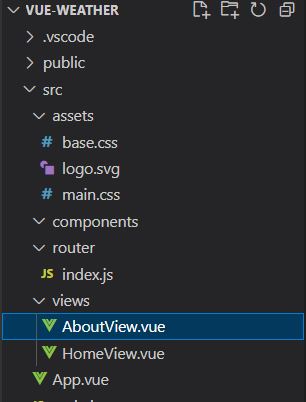
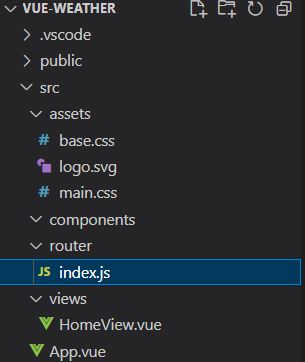
- HomeView.js
<script setup></script>
<template>
<main></main>
</template>
- App.vue
<template>
<div>
<RouterView />
</div>
</template>
<script setup>
import { RouterView } from "vue-router";
</script>
<style lang="scss" scoped></style>
Tailwind Install
- open terminal and type
npm install -D tailwindcss postcss autoprefixer
to install tailwind
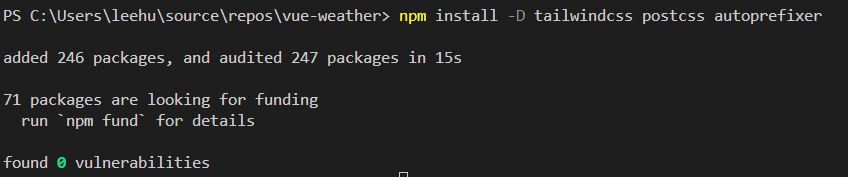
- type
npx tailwindcss init -p
to createtailwind.config.js
file which is a config for tailwindcss

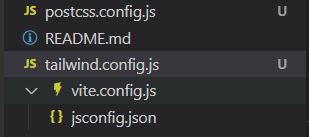
- tailwind.config.js
/** @type {import('tailwindcss').Config} */
export default {
content: ["./index.html", "./src/**/*.{vue,js,ts,jsx,tsx}"],
theme: {
extend: {
colors: {
"weather-primary": "#00668A",
"weather-secondary": "#004E71"
}
},
fontFamily: {
Roboto: ["Roboto, sans-serif"]
},
container: {
padding: "2rem",
center: true
},
screens: {
sm: "640px",
md: "768px"
}
},
plugins: []
};
-
create
tailwind.css
in assets folder -
tailwind.css
@tailwind base;
@tailwind components;
@tailwind utilities;
- main.js
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
import './assets/tailwind.css'
const app = createApp(App)
app.use(router)
app.mount('#app')
- get Roboto link in google font
-
get font-awesome link in cdnjs
- index.html
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="UTF-8">
<link rel="icon" href="/favicon.ico">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Roboto:ital,wght@0,100..900;1,100..900&display=swap" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.7.2/css/all.min.css" integrity="sha512-Evv84Mr4kqVGRNSgIGL/F/aIDqQb7xQ2vcrdIwxfjThSH8CSR7PBEakCr51Ck+w+/U6swU2Im1vVX0SVk9ABhg==" crossorigin="anonymous" referrerpolicy="no-referrer" />
<title>Vue Weather</title>
</head>
<body>
<div id="app"></div>
<script type="module" src="/src/main.js"></script>
</body>
</html>
Navigation
:to
- links with the url which is in the router
Site Navigation
-
create
SiteNavigation.vue
file in components folder -
SiteNavigation.vue
<template>
<header class="sticky top-0 bg-weather-primary shadow-lg">
<nav class="container flex flex-col sm:flex-row items-center gap-4 text-white py-6">
<RouterLink :to="{ name: 'home' }">
<div class="flex items-center gap-3">
<i class="fa-solid fa-sun text-2xl"></i>
<p class="text-2xl">The Local Weather</p>
</div>
</RouterLink>
<div class="flex gap-3 flex-1 justify-end">
<i class="fa-solid fa-circle-info text-xl hover:text-weather-secondary duration-150 cursor-pointer"></i>
<i class="fa-solid fa-plus text-xl hover:text-weather-secondary duration-150 cursor-pointer"></i>
</div>
</nav>
</header>
</template>
<script setup>
import { RouterLink } from 'vue-router';
</script>
- App.vue
<template>
<div class="flex flex-col min-h-screen font-Roboto bg-weather-primary">
<SiteNavigation/>
<RouterView />
</div>
</template>
<script setup>
import { RouterView } from "vue-router";
import SiteNavigation from "./components/SiteNavigation.vue";
</script>
<style lang="scss" scoped></style>
